Working on the LED strip. Almost done with the LED strip control.
This commit is contained in:
parent
d1c31736a3
commit
fbfcd89399
1
.gitignore
vendored
Normal file
1
.gitignore
vendored
Normal file
@ -0,0 +1 @@
|
|||||||
|
.vscode/
|
@ -1 +1,5 @@
|
|||||||
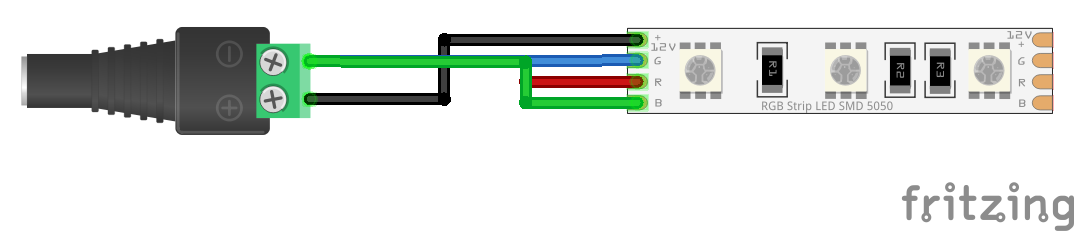
|

|
||||||
|
Source: https://randomnerdtutorials.com/esp32-esp8266-rgb-led-strip-web-server/
|
||||||
|
|
||||||
|
TODO:
|
||||||
|
* [ ] Add a relay
|
@ -1,8 +1,8 @@
|
|||||||
|
|
||||||
// Define the pins for the LED strip
|
// Define the pins for the LED strip
|
||||||
#define RED 23
|
#define RED 25
|
||||||
#define GREEN 33
|
#define GREEN 33
|
||||||
#define BLUE 26
|
#define BLUE 32
|
||||||
|
|
||||||
|
|
||||||
void setup() {
|
void setup() {
|
||||||
@ -13,29 +13,43 @@ void setup() {
|
|||||||
Serial.begin(9600);
|
Serial.begin(9600);
|
||||||
}
|
}
|
||||||
|
|
||||||
|
// Print current values of the pins
|
||||||
|
void printValues() {
|
||||||
|
Serial.println("Red: " + String(analogRead(RED)));
|
||||||
|
Serial.println("Green: " + String(analogRead(GREEN)));
|
||||||
|
Serial.println("Blue: " + String(analogRead(BLUE)));
|
||||||
|
}
|
||||||
|
|
||||||
// Function that sets the color of the LED strip
|
// Function that sets the color of the LED strip
|
||||||
void setColor(int red, int green, int blue) {
|
void setColor(int red, int green, int blue) {
|
||||||
Serial.println("Setting color to: " + String(red) + ", " + String(green) + ", " + String(blue));
|
|
||||||
|
|
||||||
|
// Set the color of the LED strip
|
||||||
|
analogWrite(RED, red);
|
||||||
|
analogWrite(GREEN, green);
|
||||||
|
analogWrite(BLUE, blue);
|
||||||
|
printValues();
|
||||||
|
return;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
void stop() {
|
||||||
|
// Function which turns off the pins
|
||||||
|
analogWrite(RED, 0);
|
||||||
|
analogWrite(GREEN, 0);
|
||||||
|
analogWrite(BLUE, 0);
|
||||||
|
|
||||||
|
Serial.println("Turning off the LED strip");
|
||||||
|
printValues();
|
||||||
|
return;
|
||||||
|
}
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
void loop() {
|
void loop() {
|
||||||
// Set the color to red
|
// Alternate between off and white over 5 seconds
|
||||||
setColor(255, 0, 0);
|
stop();
|
||||||
delay(1000);
|
delay(5000);
|
||||||
// Set the color to green
|
|
||||||
setColor(0, 255, 0);
|
|
||||||
delay(1000);
|
|
||||||
// Set the color to blue
|
|
||||||
setColor(0, 0, 255);
|
|
||||||
delay(1000);
|
|
||||||
// Set the color to white
|
|
||||||
setColor(255, 255, 255);
|
setColor(255, 255, 255);
|
||||||
delay(1000);
|
delay(5000);
|
||||||
// Set the color to off
|
|
||||||
setColor(0, 0, 0);
|
|
||||||
delay(1000);
|
|
||||||
}
|
}
|
||||||
|
|
Loading…
x
Reference in New Issue
Block a user